Python’s ecosystem offers an array of testing frameworks, but Pytest stands out for its simplicity and versatility. One of the common testing scenarios is asserting that a function was called, especially when you’re working with mocking.
Let’s embark on a journey to understand this essential feature of Pytest.
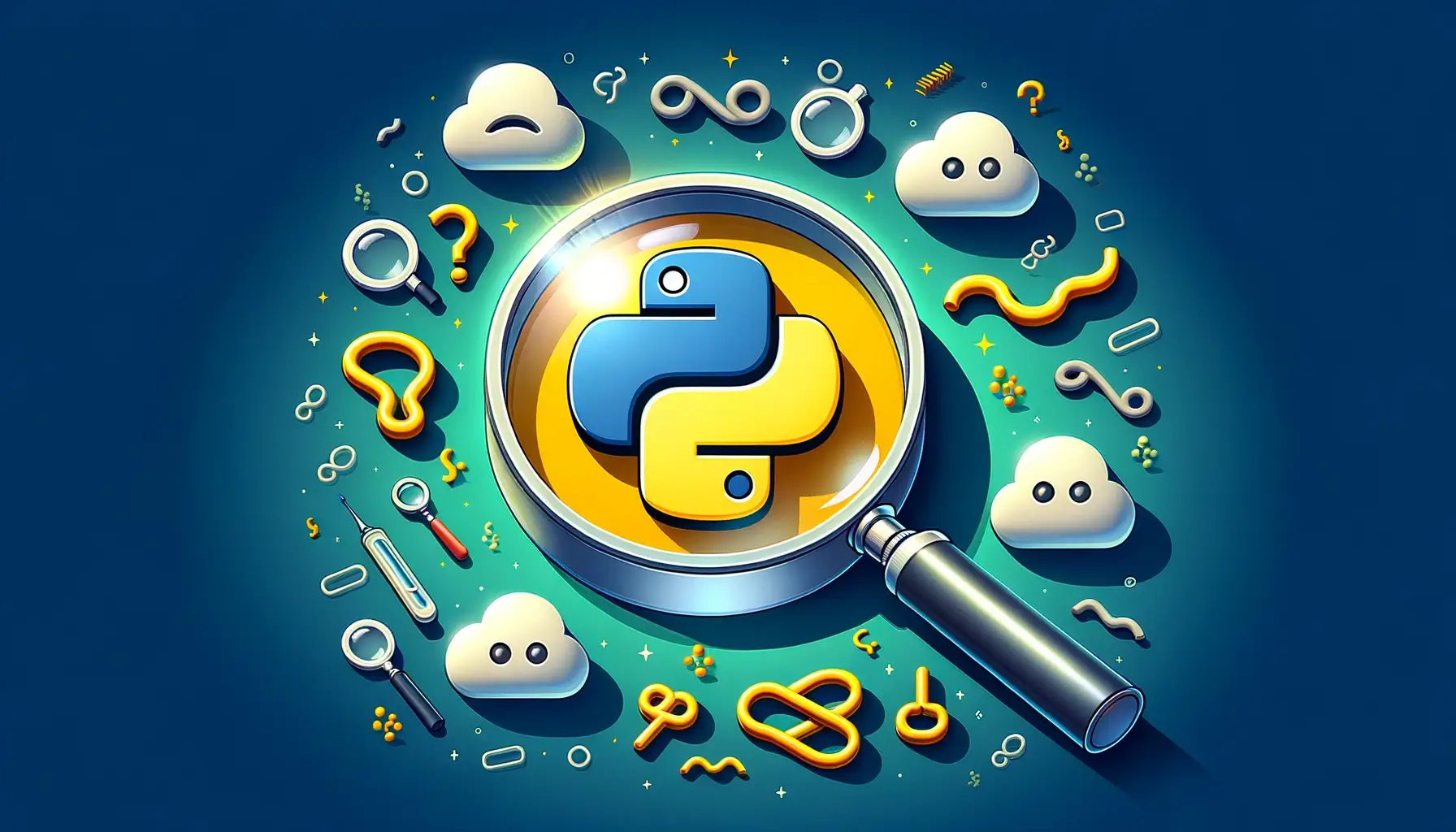
The Essence of Assertions in Pytest
Assertions play a critical role in unit testing. They allow developers to set expectations about the behavior of their code. When an assertion passes, it means your code behaves as expected, but when it fails, you’re alerted to a potential issue.
The Power of Pytest Assertions
Unlike other testing libraries, Pytest offers a more human-readable assertion syntax. Instead of using specific assert methods like assertEquals
or assertNotNone
, you can use plain assert
statements, making your tests cleaner and more intuitive.
Verifying Function Calls with Pytest
Before we plunge into the specifics of asserting function calls, it’s crucial to understand mocking. Python’s unittest.mock
provides a set of tools to replace parts of your system under test with mock objects and make assertions about how they’ve been used. When integrated with Pytest, it’s a formidable tool in a developer’s arsenal.
The Art of Asserting Function Calls
With the groundwork of mocking set, let’s explore how to assert that a function has been called:
- The Basic Check: Use
mock_object.assert_called()
to ensure your function was invoked. - Asserting Call Count: If you want to be specific about how many times a function was called,
mock_object.call_count
can be your friend. - Verifying Call Arguments: If you wish to be certain about the parameters with which your function was invoked,
mock_object.assert_called_with(arg1, arg2, ...)
is the go-to method.
Advanced Techniques in Pytest for Function Call Assertions
pytest-mock
is an elegant plugin that extends the functionalities provided by unittest.mock
. One of its notable features is mocker.spy
, which allows you to “spy” on function calls without altering their original behavior. This is exceptionally useful when you want to track function calls but also need the original function to execute.
Chaining Assertions
One of the strengths of Pytest is its ability to chain multiple assertions together, making your tests both comprehensive and clean. For instance, after verifying a function’s call, you can proceed to check the result it returned or any side effects it might have had.
Conclusion
Asserting that a function was called, or even more specifically, that it was called with the right arguments, is foundational in software testing. With tools like Pytest and unittest.mock
, Python developers have robust means to ensure their software behaves as expected, leading to more reliable and maintainable applications.
Remember, the core of effective testing isn’t just about catching bugs, but about building confidence in your software’s functionality.
Note: Always refer to the official Pytest documentation and Python’s unittest.mock documentation for the most up-to-date and accurate information.
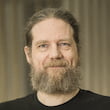
Martijn Pieters is a seasoned Principal Software Engineer and Software Architect with a rich background in crafting innovative software solutions. Beyond technical expertise, Martijn passionately mentors upcoming talents, sharing insights from his extensive experience to shape the next generation of tech leaders.