Every programmer, at some point, has faced a pesky error that seemed insurmountable. One such common Python error is the “list object has no attribute lower.”
If you’ve stumbled upon this article, chances are you’ve encountered this issue and are looking for a solution. Well, you’re in the right place!
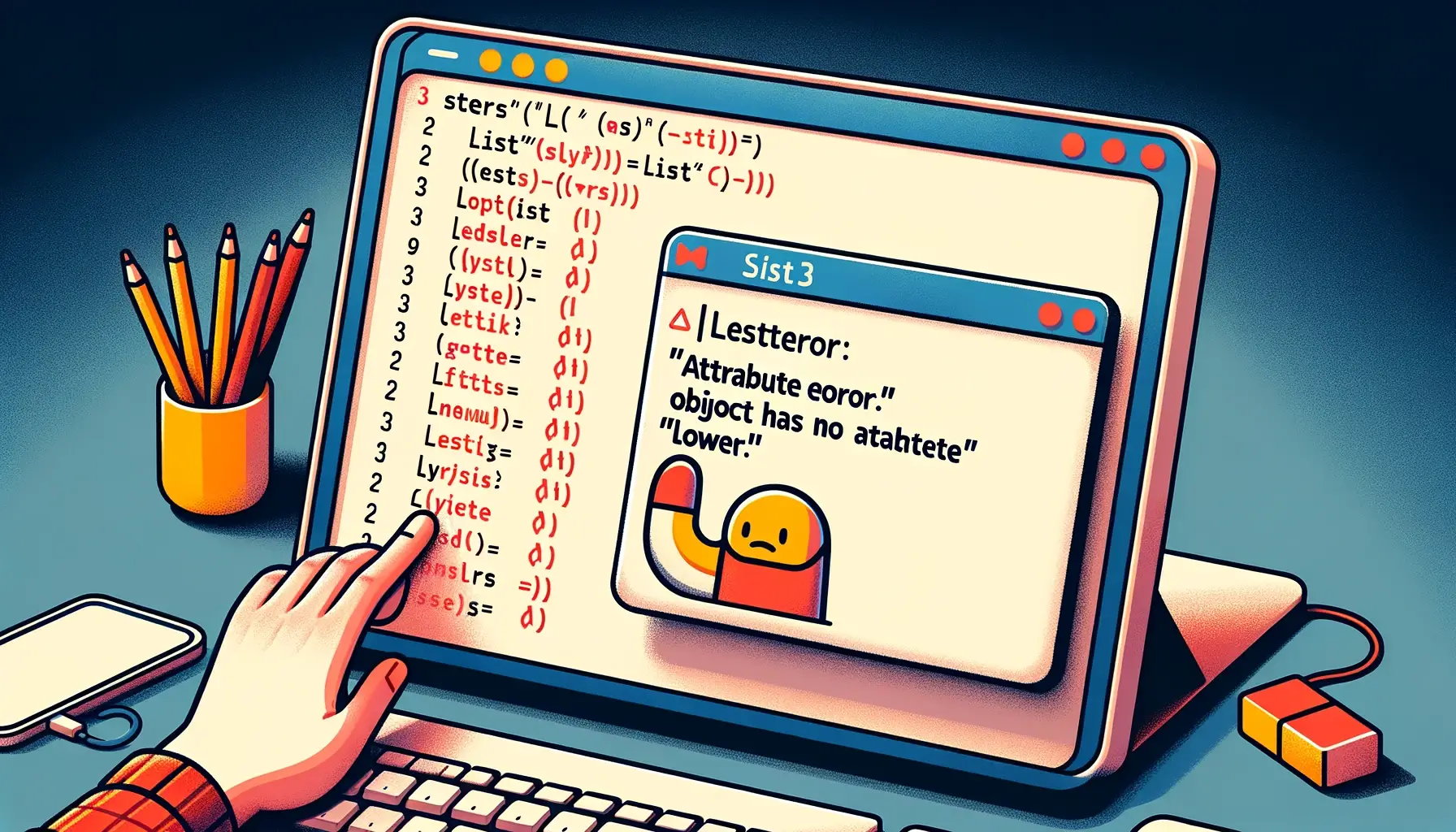
The Heart of the Matter
Before diving into the solution, it’s essential to understand the error’s root cause. In Python, every data type has attributes and methods associated with it. For instance, strings have a .lower()
method that converts all characters in the string to lowercase.
However, lists do not have this method, which leads us to the crux of the issue: trying to apply a string method to a list object.
Common Scenarios Where the Error Occurs
1. Directly Calling on a List
my_list = ["HELLO", "WORLD"] print(my_list.lower())
This will throw the error since you can’t directly call .lower()
on a list.
2. Nested Lists
my_list = [["HELLO", "WORLD"], ["PYTHON", "ROCKS"]] for sublist in my_list: print(sublist.lower())
Even if you’re iterating through the list, you’ll encounter the error when trying to apply .lower()
to a sublist, which is still a list object.
Practical Solutions to the Problem
1. Using List Comprehension: A concise and efficient way to convert each string in a list to lowercase.
my_list = ["HELLO", "WORLD"] lowercase_list = [item.lower() for item in my_list] print(lowercase_list)
2. Using a Loop: A more traditional approach, iterating through each item in the list.
my_list = ["HELLO", "WORLD"] lowercase_list = [] for item in my_list: lowercase_list.append(item.lower()) print(lowercase_list)
3. Handling Nested Lists: For nested lists, you can use nested loops or list comprehensions.
my_list = [["HELLO", "WORLD"], ["PYTHON", "ROCKS"]] lowercase_list = [[item.lower() for item in sublist] for sublist in my_list] print(lowercase_list)
Key Takeaways
- Always be aware of the data type you’re working with and the methods associated with it.
- Errors are a natural part of the coding journey. They provide opportunities to learn and grow.
- The “list object has no attribute lower” error is a reminder to apply methods to appropriate data types.
Conclusion
In conclusion, while the “list object has no attribute lower” error might seem daunting at first, understanding its cause and knowing how to address it can help you navigate Python more smoothly. Remember to always check the type of object you’re working with and use methods that are suitable for that object. Happy coding!
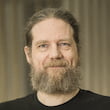
Martijn Pieters is a seasoned Principal Software Engineer and Software Architect with a rich background in crafting innovative software solutions. Beyond technical expertise, Martijn passionately mentors upcoming talents, sharing insights from his extensive experience to shape the next generation of tech leaders.