The world of programming is brimming with intricate terminologies and concepts, and one such term that often pops up, especially in the realm of file handling in Python, is _io.TextIOWrapper
. If you’ve ever tried working with files in Python, there’s a good chance you’ve come across this term.
For many, it might seem like a technical jargon that’s too complex to decode. But worry not! By the end of this blog post, you’ll have a clear understanding of what _io.TextIOWrapper
is and how to effortlessly read and print its data.

What is _io.TextIOWrapper?
Before diving into the “how-to”, let’s first grasp the “what”.
The _io.TextIOWrapper
is a part of Python’s input-output (io
) module. In simpler terms, it’s an interface or a wrapper around a buffered I/O object, responsible for encoding and decoding data into text.
Whenever you open a text file using Python’s built-in open
function without specifying a mode, by default, you get an _io.TextIOWrapper
object. It facilitates the reading and writing of strings to a file.
Steps to Read/Print the Data from _io.TextIOWrapper
- Opening a File: To start reading or printing the data, you first need to open the desired file. This can be done using the
open
function.
file_obj = open('your_file_path.txt', 'r')
Here, ‘r’ stands for read mode. If you print the type of file_obj
, it will display <class '_io.TextIOWrapper'>
.
Reading the File: Once the file is open, you can read its contents in various ways:
- Reading the Entire Content at Once:
content = file_obj.read() print(content)
Reading Line-by-Line:
for line in file_obj: print(line)
Reading a Fixed Number of Characters:
first_ten_chars = file_obj.read(10) print(first_ten_chars)
3. Closing the File: After extracting all the necessary data, ensure to close the file. It’s a best practice to free up the resources.
file_obj.close()
4. The Safer Approach – Using ‘with’ Statements: A more Pythonic way to handle files is by using the with
statement. This ensures that the file is properly closed after its suite finishes.
with open('your_file_path.txt', 'r') as file_obj: content = file_obj.read() print(content)
Why Is It Essential to Understand _io.TextIOWrapper?
Having clarity about _io.TextIOWrapper
ensures that you’re more proficient in handling text files in Python. It allows you to manipulate data seamlessly, be it reading configurations, storing results, or parsing data.
Conclusion
The beauty of programming lies in its vastness, with every concept interwoven intricately with another. While _io.TextIOWrapper
might have seemed like a convoluted term initially, breaking it down step by step makes it more comprehensible.
By understanding its essence and functionalities, you’re now one step closer to mastering the art of file handling in Python.
Note: This blog post emphasizes on Python’s file handling mechanism. To explore more about Python’s io module, you can always refer to the official Python documentation.
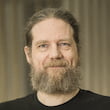
Martijn Pieters is a seasoned Principal Software Engineer and Software Architect with a rich background in crafting innovative software solutions. Beyond technical expertise, Martijn passionately mentors upcoming talents, sharing insights from his extensive experience to shape the next generation of tech leaders.